Master Browser Debugging: Unlock the Full Potential of JavaScript Console
Introduction
Debugging is something coders try to avoid, but often end up causing more mistakes. Writing code without bugs is really hard, even for experts. That's why it's important to always debug your code.
Console In Practice
The console.log()
function is not exclusive to browsers;
it is a part of the JavaScript language and can be used in various JavaScript environments,
such as Node.js as well. However, the implementation can differ depending on the environment.
In a browser, console.log()
outputs messages to the web console.
In a Node.js environment, it prints to the standard output (stdout).
Many developers use console.log()
for checking JavaScript errors, but there are better ways to do it. This article is all about showing you those better methods.
In a good code editor, just typing 'console' will give you a list of helpful options, making debugging easier.
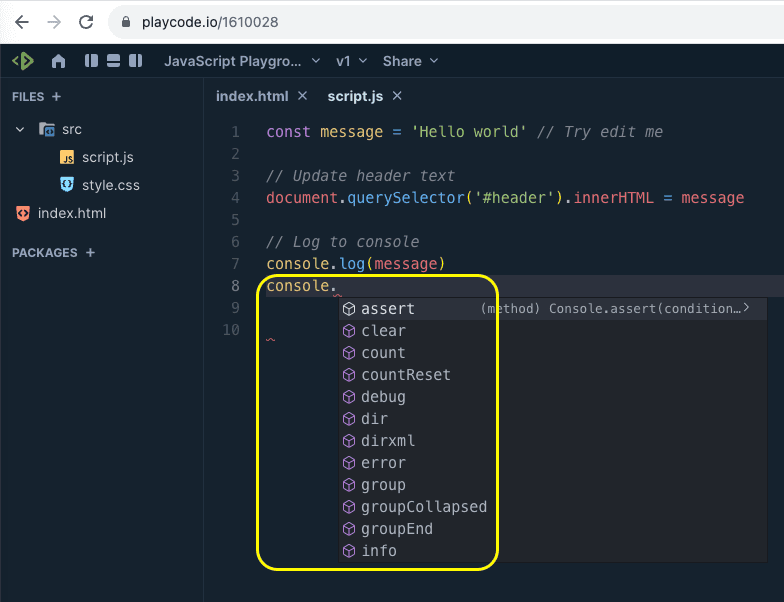
If you scroll the popup menu you will see even more options
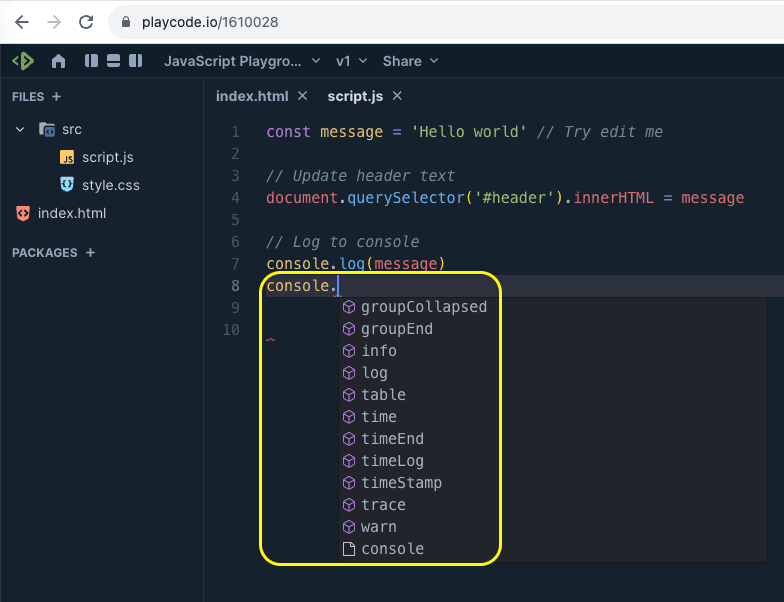
console.error() & console.warn()
Rather than sticking with the usual console.log()
,
consider these superior alternatives that can streamline and speed up your debugging process as they
make it easier to understand the code and diagnose issues.
The console.error()
and console.warn()
methods are similar to console.log()
but are
generally used to display error and warning messages, respectively.
Both methods print messages to the console,
but they also often display these messages in a way that makes it clear they represent errors or warnings.
console.error():
This function prints an error message to the console.
In most browser-based environments, it will highlight the message with a red background.
It's generally used when an operation fails or an error occurs.
console.warn():
This function prints a warning message to the console.
In most browsers, it will highlight the message with a yellow background.
This is often used to indicate a potential problem that doesn't stop
the function of the system but might do so in the future.
console.log('This is a general message.')
console.warn('This is a warning!')
console.error('An error occurred!')
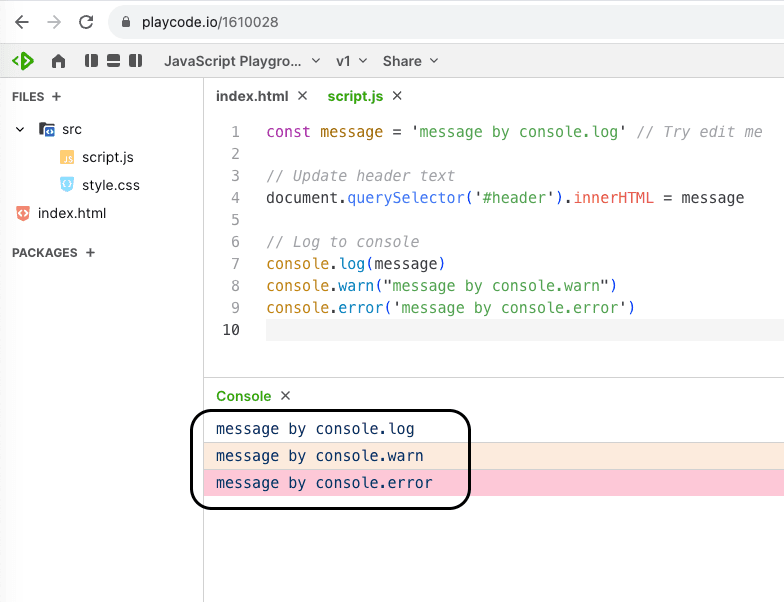
console.time()
The console.time()
method is used in JavaScript to start a timer that can be used to
track how long an operation takes to complete. The timer is uniquely identified by a label.
To stop the timer and display the time elapsed, you can use the console.timeEnd()
method,
specifying the same label. The time is usually displayed in milliseconds.
Here's a basic example:
console.time('My timer')
// some code or function you want to time
for (let i = 0; i < 1000000; i++) {
// Do nothing
}
console.timeEnd('My timer')
When you run this code, you'll get output in the console that might look something like this:
My timer: 23.12345ms
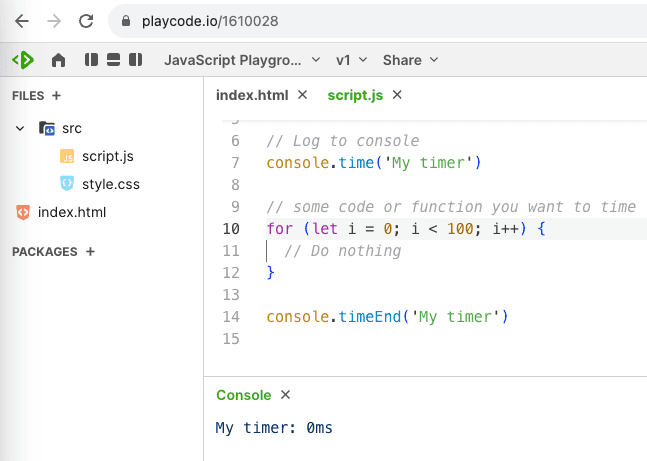
Looping through 100 iterations is not a resource-intensive task and can execute very quickly!
Note: For more complex performance profiling, like in a React project, you might want to look into more advanced tools and libraries like React Profiler or browser-based profiling tools. This way, you'll get more comprehensive insights into the performance aspects of your application.
console.trace()
The console.trace()
method outputs a stack trace to the console at the point where it is called.
A stack trace is essentially a report of the active stack frames at that point in time,
providing an overview of the execution context and the sequence of function calls that
led to the current point in the code. This can be extremely helpful for debugging,
as it shows you how your code arrived at a particular state.
Here's a basic example:
function firstFunction() {
secondFunction()
}
function secondFunction() {
thirdFunction()
}
function thirdFunction() {
console.trace()
}
firstFunction()
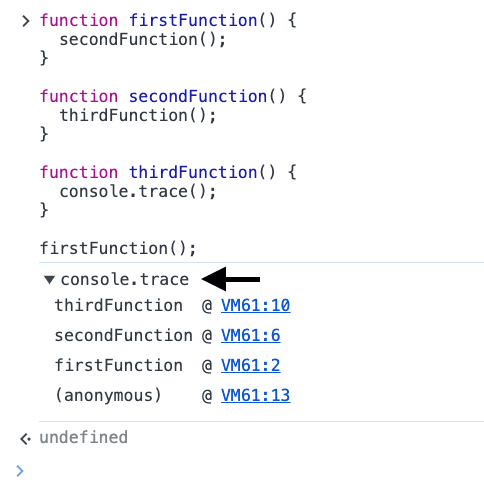
Note: anonymous means the main JavaScript code.
console.group
The console.group()
method is used to group together related log messages, making it easier to organize
output in the console. Once console.group()
is called, all subsequent console output will be indented
until console.groupEnd()
is called. This makes it easier to read and follow the flow of log messages,
especially when you have complex code that generates a lot of output.
You can also nest groups within groups for more complex hierarchical logging.
Here's a basic example:
console.group('Level 1')
console.log('This is level 1')
console.group('Level 2')
console.log('This is level 2')
console.groupEnd()
console.log('Back to level 1')
console.groupEnd()
When you run this code, you'll see the messages are neatly organized into groups in the console:
Level 1
| This is level 1
| Level 2
| This is level 2
| Back to level 1
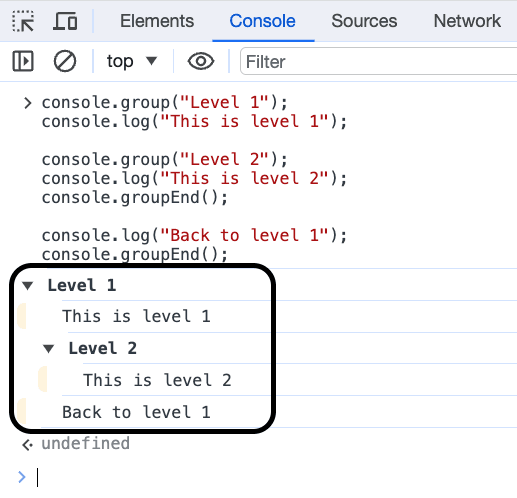
console.clear
The console.clear()
method is used to clear the console window of any existing messages,
making it easier to focus on new messages.
This can be particularly useful when debugging or during development to declutter
the console and make it easier to read subsequent messages. When you call this method,
the console is cleared, and typically,
a message like "Console was cleared" is displayed to indicate that the action was performed.
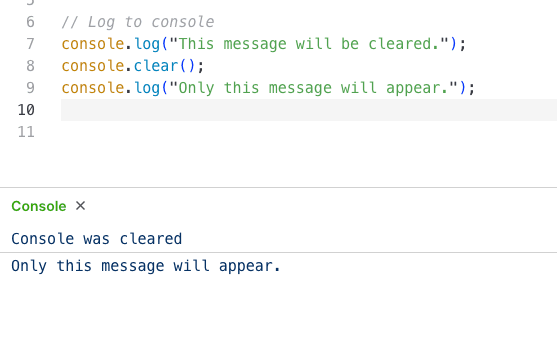
console.table
The console.table()
method displays tabular data as a table in the console.
It takes one mandatory argument, which is the data to be displayed,
usually an array or an object. Optionally, you can also specify columns to include in the table.
This makes it easier to visualize and analyze complex data structures, particularly useful during debugging.
Here's a basic example using an array of objects:
const people = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 35 },
]
console.table(people)
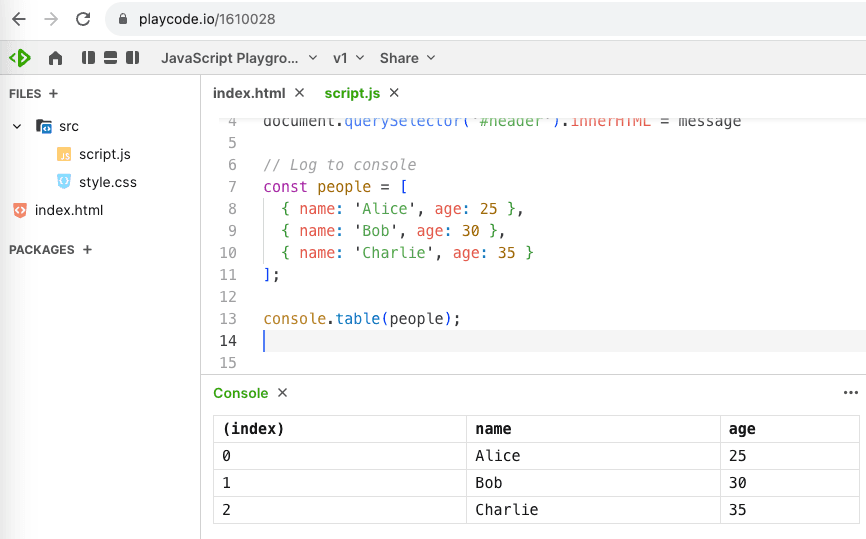
Styling console
You can style console logs in the browser by using the %c
directive within the console.log()
function.
This allows you to apply CSS styles to the console output.
You can specify the styles in the second argument as a string, which works pretty much like inline CSS.
Here's a simple example:
console.log(
'%c This is a styled message!',
'color: blue; font-size: 24px; background-color: yellow;',
)
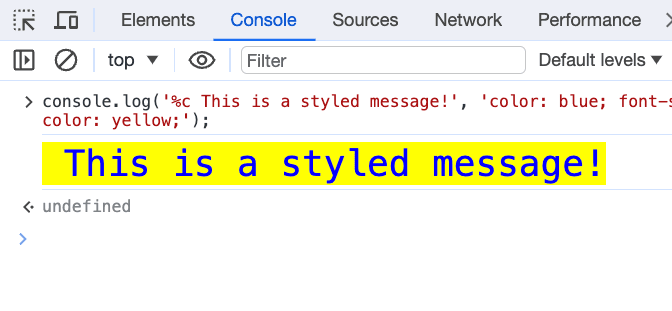
Multiple Styles:
You can also use multiple %c
directives to style different parts of a message differently:
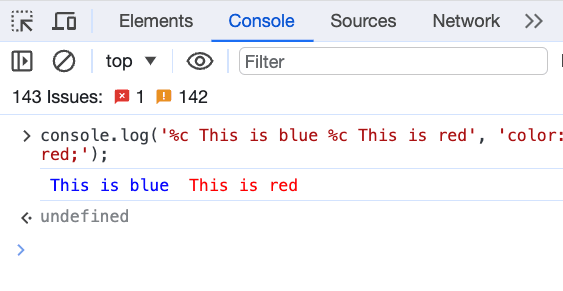
Final Note: If you find that you're using console methods frequently, especially for debugging, consider implementing a more robust logging solution (like winston or pino in a Node.js environment). This can help you better control your logging levels and keep your codebase clean, maintainable, and scalable.
Now the life is beautiful, try it out and enjoy it!